Uses for Implicitly Unwrapped Optionals in Swift
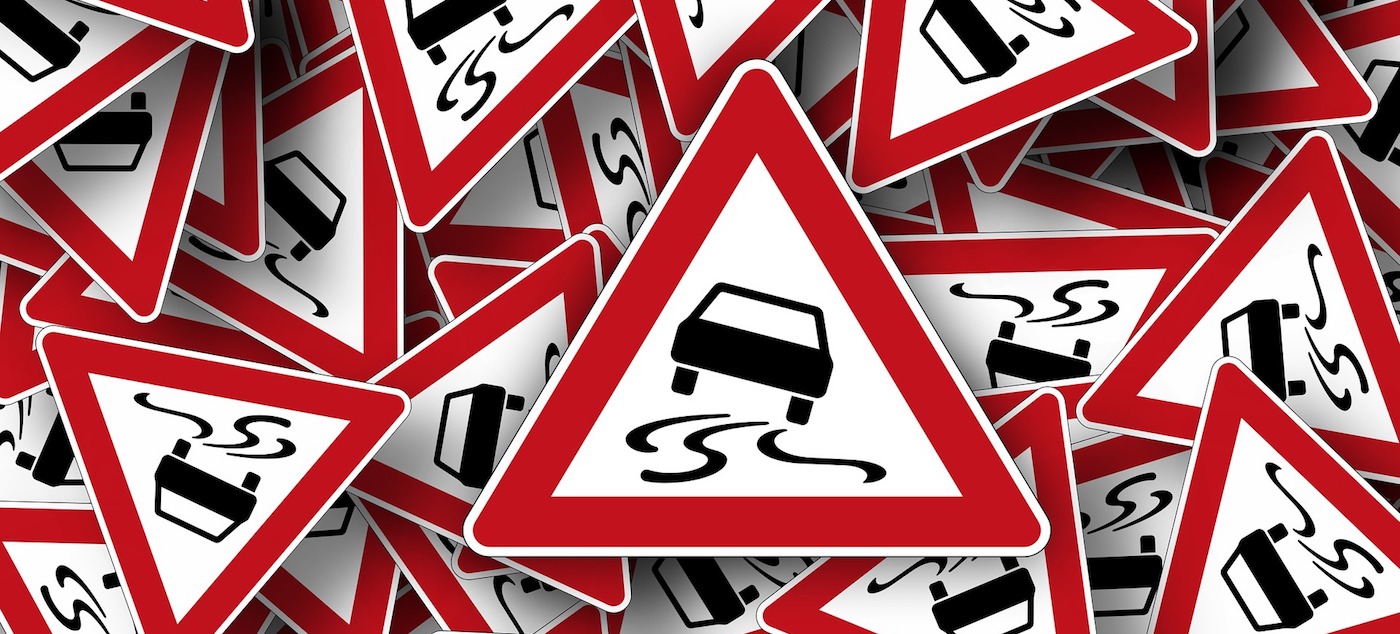
Before I can describe the use cases for Implicitly Unwrapped Optionals, you should already understand what Optionals and Implicitly Unwrapped Optionals are in Swift. If you do not, I recommend you first read my article on optionals
When To Use An Implicitly Unwrapped Optional
There are two main reasons that one would create an Implicitly Unwrapped Optional. All have to do with defining a variable that will never be accessed when nil
because otherwise, the Swift compiler will always force you to explicitly unwrap an Optional.
1. A Constant That Cannot Be Defined During Initialization
Every member constant must have a value by the time initialization is complete. Sometimes, a constant cannot be initialized with its correct value during initialization, but it can still be guaranteed to have a value before being accessed.
Using an Optional variable gets around this issue because an Optional is automatically initialized with nil
and the value it will eventually contain will still be immutable. However, it can be a pain to be constantly unwrapping a variable that you know for sure is not nil. Implicitly Unwrapped Optionals achieve the same benefits as an Optional with the added benefit that one does not have to explicitly unwrap it everywhere.
A great example of this is when a member variable cannot be initialized in a UIView subclass until the view is loaded:
class MyView: UIView {
@IBOutlet var button: UIButton!
var buttonOriginalWidth: CGFloat!
override func viewDidLoad() {
self.buttonOriginalWidth = self.button.frame.size.width
}
}
Here, we have two different implicitly unwrapped optionals. Xcode forces us to make IBOutlets optional because the cannot be set during initialization. Instead they are set while loding the view. You could use a normal optional but if the interface file is setup correctly, the value will never be nil. It can also be nice to have an interface crash on loading if you forget to connect an outlet instead of harder to debug behavior down the line.
Also, you cannot calculate the original width of the button until the view loads, but you know that viewDidLoad
will be called before any other method on the view (other than initialization). Instead of forcing the value to be
explicitly unwrapped pointlessly all over your class, you can declare it as an Implicitly Unwrapped Optional.
2. When Your App Cannot Recover From a Variable Being nil
This should be extremely rare, but if your app could literally not continue to run if a variable is nil
when accessed, it would be a waste of time to bother testing it for nil
. Normally if you have a condition that must absolutely be true for your app to continue running, you would use an assert
. An Implicitly Unwrapped Optional has an assert for nil built right into it.
When Not To Use An Implicitly Unwrapped Optional
1. Lazily Calculated Member Variables
Sometimes you have a member variable that should never be nil, but it cannot be set to the correct value during initialization. One solution is to use an Implicitly Unwrapped Optional, but a better way is to use a lazy variable:
class FileSystemItem {
}
class Directory : FileSystemItem {
@lazy var contents : [FileSystemItem] = {
var loadedContents = [FileSystemItem]()
// load contents and append to loadedContents
return loadedContents
}()
}
Now, the member variable contents
is not initialized until the first time it is accessed. This gives the class a chance to get into the correct state before calculating the initial value.
Note: This may seem to contradict #1 from above. However, there is an important distinction to be made. The buttonOriginalWidth
above must be set during viewDidLoad to prevent anyone changing the buttons width before the property is accessed.
2. Everywhere Else
For the most part, Implicitly Unwrapped Optionals should be avoided because if used mistakenly, your entire app will crash when it is accessed while nil
. If you are ever not sure about whether a variable can be nil, always default to using a normal Optional. Unwrapping a variable that is never nil
certainly doesn't hurt very much.